React.js –
It’s different !
What is react?
React js is a lightweight front end JavaScript library that is used for creating user interfaces. Sometimes referred as V in the MVC (which is a self-admission on React official documentation). React is very unique one of its vital feature is that it can be rendered on server side too, and both client-server can work together interoperable.
Some other facts about React – it was created by Facebook R&D, first released in 2013. It is used at Facebook in production, and Instagram.com is completely written in React.
Why we need something like react?
We have long way from traditions request-response model of web development. With ajaxification of apps user can’t wait for the request to go respond back and page to reload. And advent of single page apps became faster but at the same time complex. We can no longer re-render a page when a request occurs. Managing the state itself became difficult. These problems are something devs face everyday and React is the facebook/instagram team way of solving them. React philosophy greatly differs from usual frameworks. In this article we will explore how react is changing tradition way of thinking by understanding its key concepts which are disrupting conventional way of building front-end apps.
Key Concepts
Virtual DOM
Virtual DOM is a brand new concept, which abstracts away the DOM from you. Not so clear, let us go a bit deep on this feature. The problem responsible for creation of React was to render a section of the view as quickly as possible and Virtual DOM plays the significant role in helping achieveing this how? As the state changes Virtual DOM selectively renders sub-trees of nodes based upon this state change. This leads to least amount of DOM manipulation possible in order to keep your components aware of latest changes. In short react uses a fake DOM.
Lets take an example of a car which is our object, and has all features what a car must have, what Virtual dom does is it simply copies this cars current state (makes its replica). Now if we take this car object and make some changes like adding a gps system, changing its color, or a new cowboy style bumper. In react world when these changes are made two things happen – One, react runs a algorithm which recognizes that these changes have been made and what are the differences. Two, it updates the Dom with these differences. In other word it does not build the car again from ground up rather only make the required changes of color, gps and new bumper.
Before we move to next key concept let’s first install react to understand the examples more clearly.
Installation
The easiest way to start working with React is using JSFiddle examples :
React JSfiddle with JSX (we will learn about JSX shortly) -> https://jsfiddle.net/reactjs/69z2wepo/
React JSfiddle > https://jsfiddle.net/reactjs/5vjqabv3/
Other way is to download complete starter kit to even work offline
http://facebook.github.io/react/downloads/react-0.13.3.zip
You may also install react-tools this required npm :
npm install -g react-tools
Another usefull instrument to boost your productivity with React is React developer tools. Which is a very useful chrome extension that allows you to inspect React component hierarchy in chrome browser.
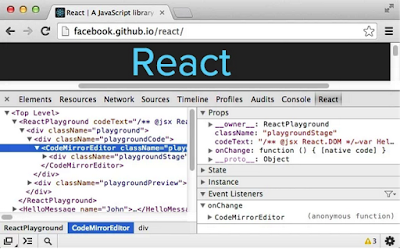
Before we dwelve into next section its time for “Hello World” program. Lets create a ‘helloworld.html’
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | <!DOCTYPE html> <html> <head> <script src=”build/react.js”></script> <script src=”build/JSXTransformer.js”></script> </head> <body> <div id=”intro”></div> <script type=”text/jsx”> React.render( <h1>Hello, world </h1>, document.getElementById(intro) ); </script> </body> </html> |
If you have download reactjs version from github you will find react.js & JSXTransformer.js in build folder. Else, you can simple refer to following urls
react.js : http://fb.me/react-0.13.3.js
JSXtransformer : http://fb.me/JSXTransformer-0.13.3.js
That was simple nothing much to explain except the keyword ‘React’ which is the entry point in to react library. Also, this react code can live in a separate file and can be referenced in the html. Lets create a separate file ‘src/helloworld.js’
1 2 3 4 | React.render( <h1>Hello, world </h1>, document.getElementById(intro) ); |
And reference in our ‘helloworld.html.’
1 | <script type=”text/jsx” src=”src/helloworld.js”></script> |
You can use simple python server to run your Hello World example
($ python -m SimpleHTTPServer)
Now that we are all setup let us looks at some other concepts of react.
JSX
JSX stands for JavaScript XML syntax transform and what does it do it lets you write HTMLtype tags in your JavaScript. In other words React uses a specified version of JavaScript called jsx. Whose basic notion is to provide intuitive syntactic sugar, which represents react internal version of the DOM and is used by react components (which are the building blocks of a react app). You are fundamentally just writing XML object representations.
We must note that JSX should eventually be converted into regular JavaScript. This can be done in few ways first one is include JSXTransformer.js in your project. Other way is to precompile JSX code beforehand. This we can do using command like tools. If we have already installed react-tools using npm as mentioned in last section simply run following command :
jsx –watch src/ build/
Components
In a react project components are the building blocks of your application. Components are modular, independent, dynamic representations of HTML in your application. Most of the times Components are children of other react Components.
Let us understand this with an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | /** @jsx React.DOM */ var car = React.createClass({ render: function() { return ( <p>{this.props.name}</p> ); } }); var Carlist = React.createClass({ render: function() { return ( <h1>My Cars:</h1>, <car name=”Mercedes /> ); } }); React.renderComponent( <Carlist />, document.querySelector(‘body’) ) |
As you see in this example there is a renderComponent what this does is it kicks off the application by rendering a component into the DOM node. You must be wondering what is this syntax I am looking at is it HTML nope its JSX (which we had discussed in our last section), which may look like HTML but is not representing actual DOM. This is reacts syntactic sugar which help us interact with virtual dom.
So far we see react is changing the way we work in front-end application. Its very different then other popular frameworks like Angular, backbone, ember and it wont be justice to them comparing it to react – as both have difference use cases. The only difference between react and other popular frameworks is that React expects you of getting and pushing data from the server. Which makes React very easy to implement front-end solution, since it simply expects you to hand it data rest all is handled by React.
BTW Who are using it ?
To build your confidence in this technology lets look at some popular apps who are using React in their production.
Air bnb
Yahoo
And many more…
We live in an era with so many js frameworks, no month passes by that we don’t find a new js framework to test. Sometimes its hard to find which framework suits our requirements. But react seems worth spending the time, for starters you may just include it to build some component in your existing application coz its light weight it wont impact your app and gradually you may convert your complete with React components.
Further Reading
React community is evolving very quickly every other week you find something new created. You may further read about Flux (application architecture which uses React views), React-Native (for building native user interface using React), Relay (framework from Facebook that provides data-fetching functionality for React apps).
Read this article and more in June 2015 edition of HealthyCode Magazine at http://healthycodemagazine.com/