In this post we will create a shell for loading any url in WebView with React-Native. Lets begin with generating the application structure :
$ react-native init WebviewShell
This will generate all necessary files for a iOS application. You can test this by building this application via Xcode.
Next, open up file index.ios.js and replace its code with following the following one :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | ‘use strict’; var React = require(‘react-native’); var { AppRegistry, StyleSheet, Text, View, WebView } = React; var WebviewShell = React.createClass({ render: function() { return ( <View style={styles.container}> <WebView url={‘https://www.facebook.com/‘} /> </View> ); } }); var styles = StyleSheet.create({ container: { flex: 1 } }); AppRegistry.registerComponent(‘WebviewShell’, () => WebviewShell); |
Let us see what we have done here. We have created a component WebviewShell which returns a View. This view returns a WebView which loads our desired url :
1 2 3 4 5 6 7 8 9 | var WebviewShell = React.createClass({ render: function() { return ( <View style={styles.container}> <WebView url={‘https://www.facebook.com/‘} /> </View> ); } }); |
When we build our project in xcode it looks something like this :
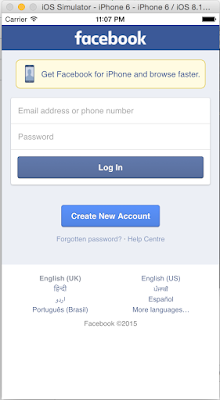
Source code for this project is available at https://github.com/akshatpaul/react-examples/tree/master/WebviewShell
Exhaustive documentation on this topic is available at React-native docs https://facebook.github.io/react-native/docs/webview.html#content