To begin using react on server side we need two things express & react, Lets setup our project :
$ npm install express react
Once this is done, next lets create two files one for our react components(component.js) and second one will be the setup file for our express server(express.js).
In component file lets create a simple component :
var React = require('react');
var Component = React.createClass({
render: function() {
return React.createElement('h1', null, this.props.msg);
}
});
module.exports = Component;
This component does nothing much simply returns h1 tag, has no props and its inner html is this.props.msg.
Next, in our express.js file lets require express, react and our component :
var express = require('express');
var React = require('react');
var Component = React.createFactory(require('./component'));
If you notice our component is wrapped in a React.createFactory() which will turn our component into a function where we can pass our props 🙂
Next, we will instantiate our express and add some routes and its corresponding function :
var app = express();
function main(request,response) {
var msg = request.params.msg || 'Hellooo';
var comp = Component({msg:msg});
response.send(comp);
}
app.get('',main);
app.get('/:msg',main);
Finally we will set it up to listen on port 4000 :
app.listen(4000)
Before explaing what is happening in home function lets fire up our terminal and run our application:
$ nodemon express.js
You will get following result on your browser :
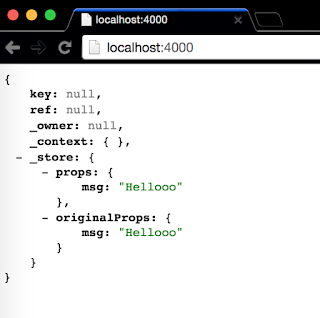
What we got here is a json representation of our component. To get our component rendered properly is by modifying our code by passing the component to React.renderToString :
function main(request,response) {
var msg = request.params.msg || 'Hi from server';
var comp = Component({msg:msg});
response.send(React.renderToString(comp))
}
Lets try out now :
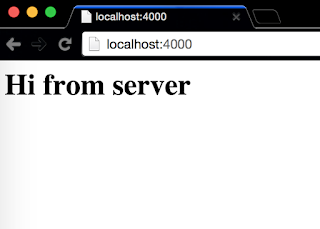
Lets pass a message parameter too http://localhost:4000/YooHoo :
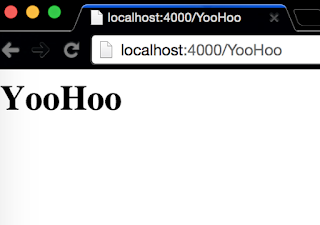
Also, if you look at your developer tools you will find all react data attributes assigned to this element:
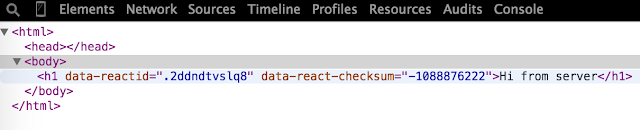
You can find this code at github :https://github.com/akshatpaul/react-examples/tree/master/react-server-side